Career Bootcamp
Resources
Recordings – (You can request an access if missing)
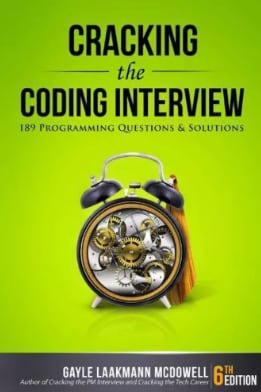
Graphs
Here are some video for you to check out:
- Algorithms Course – Graph Theory Tutorial from a Google Engineer (for the next week you only need first 1,5h of the video)
- Graph Algorithms for Technical Interviews (full course)
Week 1: (good visualization and explanation from BlueBrown and MIT):
- 3Blue1Brown – Introduction to Graph Theory: A Computer Science Perspective (good video to watch instead of MIT 6.046J)
- 3Blue1Brown – Breadth First Search (BFS): Visualized and Explained
- 3Blue1Brown – Depth First Search (DFS) Explained: Algorithm, Examples, and Code
- MIT 6.042J – Mathematics for Computer Science (this is a loooooong and lemma/proof videos)
- MIT 6.006 – Introduction to Algorithms–(another long session for those who’s interested in details and underlying proofs)
Week 2:
- FreeCodeCamp – Quick refresh on Graphs and pseudo code (the material starts on Dijkstra Algorithm)
- Algorithms by Robert Sedgewick (this is overall the best course on algorithms, for our next lesson you will need first 6 videos only for Union-Find)
Binary Trees
To read:
- Introduction to Data Structure – Binary Tree (Leetcode)
- Binary Search Tree (better to read/watch all 4 (starting from Introduction To Tree Data Structure) to understand the difference between Trees)
- Traverse a Tree – Introduction (Leetcode)
- All articles on Tree based DSA (I)
- How to implement a breadth-first search in Python (Educative.io)
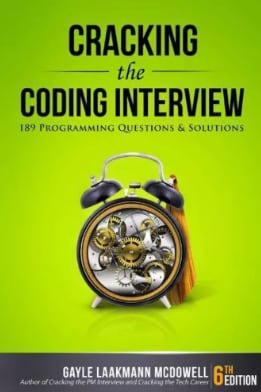
Additional:
- Week 2. Introduction to Tree Structures (Coursera)
- Binary Trees in Python (Educative.io)
- Python Data Structure and Algorithms Tutorial (Tutorialspoint)
To watch:
- Binary Search Trees, BST Sort (MIT OpenCourseWare)
- Level Order Binary Tree Traversal (Geeksforgeeks)
# | Problem | Level |
---|---|---|
1 | 104. Maximum Depth of Binary Tree | Easy |
2 | 572. Subtree of Another Tree | Easy |
3 | 112. Path Sum | Easy |
4 | 111. Minimum Depth of Binary Tree | Easy |
5 | 404. Sum of Left Leaves | Easy |
6 | 235. Lowest Common Ancestor of a Binary Search Tree | Easy |
7 | 101. Symmetric Tree | Easy |
8 | 700. Search in a Binary Search Tree | Easy |
9 | 257. Binary Tree Paths | Easy |
10 | 637. Average of Levels in Binary Tree | Easy |
11 | 100. Same Tree | Easy |
12 | 108. Convert Sorted Array to Binary Search Tree | Easy |
13 | 653. Two Sum IV - Input is a BST | Easy |
Linked Lists
To read:
- Using the Linked List Data Structure in Python
- Examples: Single Linked Lists
- 4 Incredibly Useful Linked List Logic for Interview (a summary of the different approaches to linked list leetcode problems)
To watch:
# | Problem | Level |
---|---|---|
1 | 21. Merge Two Sorted Lists | Easy |
2 | 141. Linked List Cycle | Easy |
3 | 86. Partition List | Medium |
4 | 876. Middle of the Linked List | Easy |
5 | 206. Reverse Linked List | Easy |
6 | 234. Palindrome Linked List | Easy |
7 | 160. Intersection of Two Linked Lists | Easy |
8 | 83. Remove Duplicates from Sorted List | Easy |
9 | 19. Remove Nth Node From End of List | Medium |
10 | 2. Add Two Numbers | Medium |
11 | 328. Odd Even Linked List | Medium |
12 | 138. Copy List with Random Pointer | Medium |
13 | 23. Merge k Sorted Lists | Hard |
Sliding Window
To read:
To watch:
# | Problem | Level |
---|---|---|
1 | 643. Maximum Average Subarray I | Easy |
2 | 904. Fruit Into Baskets | Medium |
3 | 1004. Max Consecutive Ones III | Medium |
4 | 1876. Substrings of Size Three with Distinct Characters | Easy |
5 | 219. Contains Duplicate II | Easy |
6 | 209. Minimum Size Subarray Sum | Medium |
7 | 1358. Number of Substrings Containing All Three Characters | Medium |
8 | 3. Longest Substring Without Repeating Characters | Medium |
9 | 340. Longest Substring with At Most K Distinct Characters | Medium |
10 | 1234. Replace the Substring for Balanced String | Medium |
11 | 567. Permutation in String | Medium |
12 | 438. Find All Anagrams in a String | Medium |
13 | 76. Minimum Window Substring | Hard |
Bit Manipulation
To read:
To watch:
# | Problem | Level |
---|---|---|
1 | 268. Missing Number | Easy |
2 | 338. Counting Bits | Easy |
3 | 137. Single Number II | Medium |
4 | 260. Single Number III | Medium |
5 | 1290. Convert Binary Number in a Linked List to Integer | Easy |
6 | 231. Power of Two | Easy |
7 | 201. Bitwise AND of Numbers Range | Medium |
8 | 461. Hamming Distance | Easy |
9 | 371. Sum of Two Integers | Medium |
10 | 1400. Construct K Palindrome Strings | Medium |
Dynamic Programming
To read:
- Cracking the Coding Interview, p.130
- Dynamic Programming: From Novice to Advanced
- DP Patterns
- A graphical introduction to dynamic programming
- Tabulation vs Memoization
- How to approach most of DP problems.
- DP (Programiz.com)
- Demystifying Dynamic Programming
To watch:
- Algorithms: Solve 'Recursive Staircase' Using Recursion (Gayle Laakmann McDowell)
- Algorithms: Memoization and Dynamic Programming (Gayle Laakmann McDowell)
- Algorithms: Solve 'Coin Change' Using Memoization and DP (Gayle Laakmann McDowell)
- Video from MIT 6.006 Introduction to Algorithms
- DP (Russian language)
Courses:
- DP with examples
- Grokking DP (Educative.io)
- Module 3 (Stepic.org, Russian language)
- Dynamic Programming - Learn to Solve Algorithmic Problems & Coding Challenges (5 hour course)
# | Problem | Level | |
---|---|---|---|
Week 1 | |||
1 | 746. Min Cost Climbing Stairs | Easy | |
2 | 70. Climbing Stairs | Easy | |
3 | 983. Minimum Cost For Tickets | Medium | |
4 | 198. House Robber | Medium | |
5 | 213. House Robber II | Medium | |
6 | 322. Coin Change | Easy | |
7 | 119. Pascal's Triangle II | Easy | |
8 | 300. Longest Increasing Subsequence | Medium | |
9 | 121. Best Time to Buy and Sell Stock | Easy | |
10 | 509. Fibonacci Number | Easy | |
11 | 122. Best Time to Buy and Sell Stock II | Medium | |
Week 2 | |||
1 | 392. Is Subsequence | Easy | |
2 | 1143. Longest Common Subsequence | Medium | |
3 | 64. Minimum Path Sum | Medium | |
4 | 63. Unique Paths II | Medium | |
5 | 787. Cheapest Flights Within K Stops | Medium | |
6 | 139. Word Break | Medium | |
7 | 416. Partition Equal Subset Sum | Medium | |
8 | 300. Longest Increasing Subsequence | Medium | |
9 | 1155. Number of Dice Rolls With Target Sum | Medium | |
10 | 518. Coin Change 2 | Medium | |
11 | 516. Longest Palindromic Subsequence | Medium |